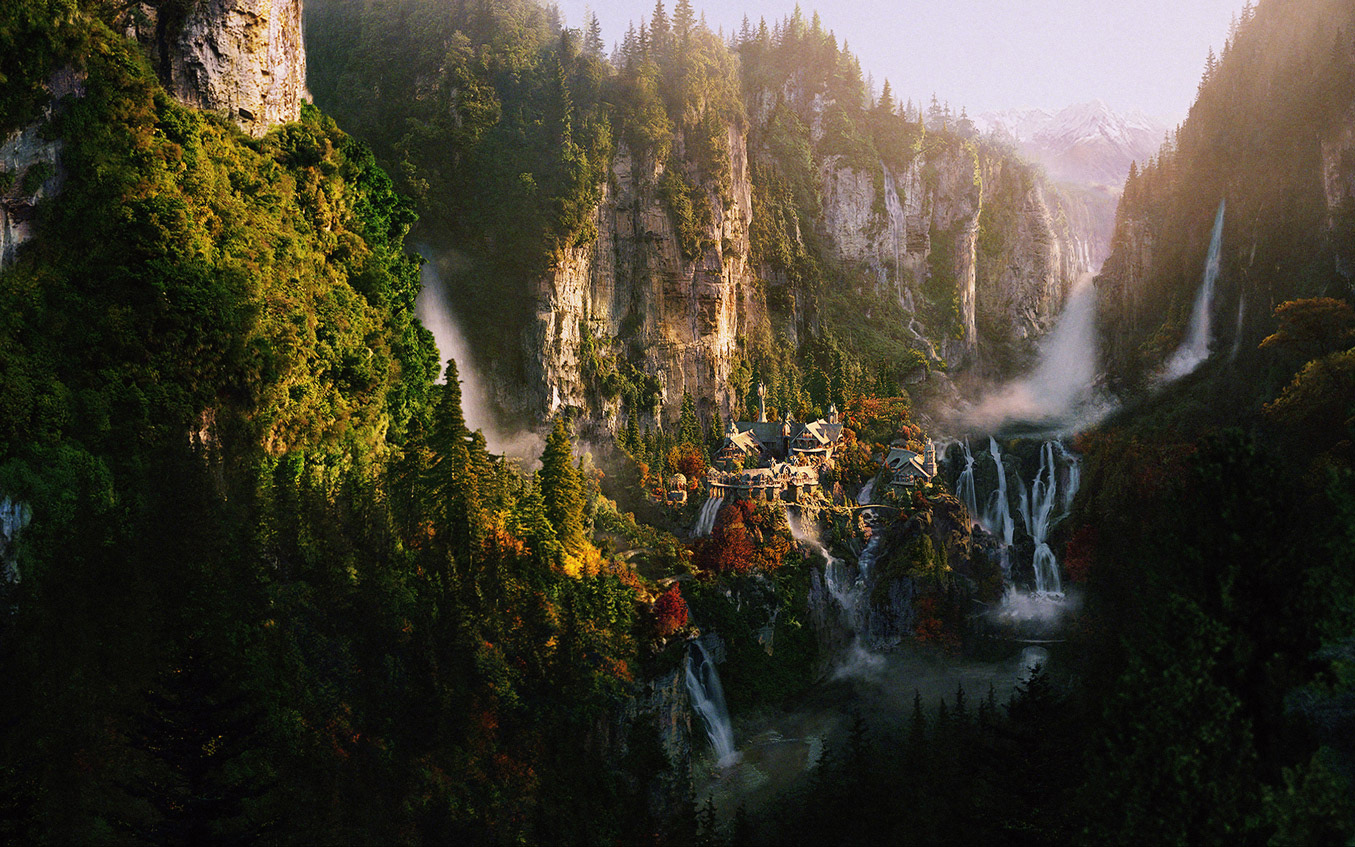
Overview
As part of a semester long project for Scripting for Interactive Digital Media I at Drexel university, I was tasked with creating a zodiac sign generator that was styled after a subject of interest to me: I chose The Lord of the Rings. This was the first project where I coded with Javascript, and at the time I was still very limited in my knowledge of coding with HTML and CSS. Aspects of the course taught me to explore the client-side scripting language that is JS. The project was both fun and also a bit frustrating at times; I discovered very quickly that JS is a whole different animal when compared to coding with HTML and CSS.
Context and Challenges
Most common zodiac generators are designed reveal to the user their astrological sign. However, the idea behind The Lord of the Rings Zodiac Generator was to reveal to the user, what character from the film franchise best describes them. So over the course of 10 weeks, I worked with various in-class examples that were designed to help me work towards the goal of having a working webpage that would host my zodiac generator.
Obviously one of the biggest challenges I faced when starting project was that JS was foreign to me; and for me to successfully complete this zodiac, I had to become familiar with how to create variables, functions and if statements. More importantly, I had to make sure that my code was clean; as I learned very quickly that if even one line of code is off in JS, then it can break your page completely. I spent many hours debugging code, and tracing my steps to see what I missed along the way.
Process and Insight
HTML Code Sample
Using the knowledge I had in HTML and CSS, I began constructing the webpage. I first started with coding in HTML setting up the basic code requirements for any webpage: <!DOCTYPE html>, <html>, <head>, and <body> tags. From there I thought about all the other pieces of code I would need to complete this pr
oject. I would certainly containers/wrappers ( or <div> tags) for each the various sections of the page. I would need <img> tags as I would be pulling in images of the characters from the films; and I would also need to utilize the <audio> tag to feature some type of spoken dialogue the chosen character. Finally, I would need to create a form so that the user could input the required data, such as their name and birthdate.
Once those element requirements were established I would need use create a style sheet and link it to the page via the <style> tag, and link the page to my JS code (after all, this was a javascript class). Check out the resulting HMTL code below.
<!DOCTYPE html> <html> <!-- NOTE: Modern browsers support HTML5 form auto checks on DATE input. In Chrome browser, try entering an invaild date i.e. Feb 31, any year. --> <head> <title>The Zodiac of Middle Earth</title> <!-- ______ ____ _ _ _______ _____ | ____/ __ \| \ | |__ __/ ____| | |__ | | | | \| | | | | (___ | __|| | | | . ` | | | \___ \ | | | |__| | |\ | | | ____) | |_| \____/|_| \_| |_| |_____/ --> <style type="text/css"> @font-face { font-family: "Ringbearer"; src: url("fonts/RINGM.TTF"); } @font-face { font-family: "Aniron"; src: url("fonts/anirb.ttf"); } @font-face { font-family: "Book Antiqua"; src: url("fonts/ANTQUAB.TTF"); } @font-face { font-family: "MorrisRoman-Black"; src: url("fonts/MorrisRoman-Black.ttf"); } </style> <!-- _____ _____ _____ / ____|/ ____/ ____| | | | (___| (___ | | \___ \\___ \ | |____ ____) |___) | \_____|_____/_____/ --> <link rel="stylesheet" href="css/main.css" /> </head> <body class="whiteScreen" onload="fade2Content();"> <!-- _____ _ _ / ____| | | (_) | | ___ _ __ | |_ __ _ _ _ __ ___ _ __ | | / _ \| '_ \| __/ _` | | '_ \ / _ \ '__| | |___| (_) | | | | || (_| | | | | | __/ | \_____\___/|_| |_|\__\__,_|_|_| |_|\___|_| --> <div id="container"> <h1>Welcome to Middle Earth</h1> <!-- _____ _ ____ |_ _| | | | _ \ | | _ __ _ __ _ _| |_| |_) | _____ __ | | | '_ \| '_ \| | | | __| _ < / _ \ \/ / _| |_| | | | |_) | |_| | |_| |_) | (_) > < |_____|_| |_| .__/ \__,_|\__|____/ \___/_/\_\ | | |_| --> <div id="rowReverse"> <div id="wrapper" style="background-color:rgba(0, 0, 0, 0.20);"> <h2>Choose your Destiny</h2> <form onsubmit="return calZodiac();"> <p> <input id="fname" name="fname" type="text" placeholder="First Name" required> </p> <input id="bday" name="bday" type="date" required> <p> <input type="submit"> </p> </form> <div id="displayMsg"></div> </div> <!-- InputBox End --> <div id="allpics"> <img class="display" src="ZodiacImages/blank.png" style="width: 230px; height: auto;" alt="blank"> <img src="ZodiacImages/aragorn.jpg" style="width: 248px; height: auto;" alt="aragorn"> <img src="ZodiacImages/faramir.jpg" style="width: 248px; height: auto;" alt="faramir"> <img src="ZodiacImages/gimli.jpg" style="width: 248px; height: auto;" alt="gimli"> <img src="ZodiacImages/frodo.jpg" style="width: 248px; height: auto;" alt="frodo"> <img src="ZodiacImages/boromir.jpg" style="width: 248px; height: auto;" alt="boromir"> <img src="ZodiacImages/pippin.jpg" style="width: 248px; height: auto;" alt="pippin"> <img src="ZodiacImages/theoden.jpg" style="width: 248px; height: auto;" alt="theoden"> <img src="ZodiacImages/samwise.jpg" style="width: 248px; height: auto;" alt="samwise"> <img src="ZodiacImages/gandalf.jpg" style="width: 248px; height: auto;" alt="gandalf"> <img src="ZodiacImages/legolas.jpg" style="width: 248px; height: auto;" alt="legolas"> <img src="ZodiacImages/merry.jpg" style="width: 248px; height: auto;" alt="merry"> <img src="ZodiacImages/treebeard.jpg" style="width: 248px; height: auto;" alt="treebeard"> </div> <div id="alltxt"> <!-- All content written on JavaScript Page --> </div> <!-- alltxt End --> </div> <!-- _ _____ _ /\ | | / ____(_) / \ ___| |_ _ __ ___| (___ _ __ _ _ __ / /\ \ / __| __| '__/ _ \\___ \| |/ _` | '_ \ / ____ \\__ \ |_| | | (_) |___) | | (_| | | | \ /_/ \_\___/\__|_| \___/_____/|_|\__, |_| |_| __/ | |___/ --> <div class="row1"> <ul> <li> <div id="aragorn">Agragon</div> </li> <li> <div id="faramir">Faramir</div> </li> <li> <div id="gimli">Gimli</div> </li> <li> <div id="frodo">Frodo</div> </li> <li> <div id="boromir">Boromir</div> </li> <li> <div id="pippin">Pippin</div> </li> </ul> </div> <div class="row2"> <ul> <li> <div id="theoden">Theoden</div> </li> <li> <div id="samwise">Samwise</div> </li> <li> <div id="gandalf">Gandalf</div> </li> <li> <div id="legolas">Legolas</div> </li> <li> <div id="merry">Merry</div> </li> <li> <div id="treebeard">Treebeard</div> </li> </ul> </div> <!--Atrosign End --> <!-- _ _ _ | | | | | | | |__| | ___| |_ __ | __ |/ _ \ | '_ \ | | | | __/ | |_) | |_| |_|\___|_| .__/ | | |_| --> <div class="popup" onclick="myFunction()">Help <span class="popuptext" id="myPopup"> How to ...<p> <img src="ZodiacImages/helpScreen.jpg" alt="helpScreen" style="max-width:550px; height:auto;"> </span>Lord of the Rings was created by J.R.R Tolkien, The film adaptations were released by New Line Cinema in 2001, 2002 and 2003. All information on this page is courtesy of lordoftherings.net. </div> <!-- Help --> </div> <!-- Container End --> <!-- Load audio files (Loook up Control preload) --> <audio id="aragornAudio" src="AudioFiles/aragornAudio.wav">Your sorry browser does notsupport HTML5 Audio!</audio> <audio id="faramirAudio" src="AudioFiles/faramirAudio.mp3"></audio> <audio id="gimliAudio" src="AudioFiles/gimliAudio.mp3"></audio> <audio id="frodoAudio" src="AudioFiles/frodoAudio.wav"></audio> <audio id="boromirAudio" src="AudioFiles/boromirAudio.wav"></audio> <audio id="pippinAudio" src="AudioFiles/pippinAudio.wav"></audio> <audio id="theodenAudio" src="AudioFiles/theodenAudio.mp3"></audio> <audio id="samwiseAudio" src="AudioFiles/samwiseAudio.mp3"></audio> <audio id="gandalfAudio" src="AudioFiles/gandalfAudio.wav"></audio> <audio id="legolasAudio" src="AudioFiles/legolasAudio.mp3"></audio> <audio id="merryAudio" src="AudioFiles/merryAudio.wav"></audio> <audio id="treebeardAudio" src="AudioFiles/treebeardAudio.wav"></audio> <!-- _ _____ | |/ ____| | | (___ _ | |\___ \ | |__| |____) | \____/|_____/ --> <script src="js/main.js"></script> </body> </html>
CSS Code Sample
While I was developing the HTML page, I was also building the my stylesheet. My main focus was to create a page with a dynamic centered appearance. By linking the sheet to my HTML page, I was able to customize previously mentioned tags; this included manipulation of the page layout, and the ability to present custom colors and fonts. You can see from the code below what some of the changes included.
/* CSS for adding content example */ * { box-sizing: border-box; } body { background-repeat: no-repeat; margin: 12px; background-color: black; /* transition on change */ opacity: 1; transition: 2s opacity; } body.whiteScreen { opacity: 0; transition: none; } #container { background-image: url(../ZodiacImages/background.jpg); background-color: none; width: 800px; height: 600px; margin: auto; border: auto; padding: auto; position: center; } #rowReverse { display: flex; justify-content: space-between; flex-direction: row; } #wrapper { background-color: black; font-family: Ringbearer, serif; font-size: 100%; color: white; max-width: 250px; padding: 5px 5px 0px 5px; margin: 15px 15px 15px 15px; border-radius: 5px; box-shadow: 1px 1px 5px black; } input { font-family: MorrisRoman-Black, serif; font-size: 100%; border: 10px none; border-radius: 10px; padding-left: 5px; padding-right: 5px; } #allpics { margin: 15px; margin-right: 260px; } #allpics img { position: absolute; transition: opacity 1s ease-in-out; opacity: 0; border-radius: 5px; } #allpics img.display { opacity: 1; } #alltxt { transition: opacity 1s ease-in-out; opacity: 1; background-color: rgba(0, 0, 0, .15); border-radius: 5px; box-shadow: 2px 2px 5px black; text-shadow: 1.5px 1.5px 1.5px black; font-family: MorrisRoman-Black, serif; color: white; font-size: 115%; padding: 5px 5px 0px 5px; margin: 15px 15px 15px 15px; width: 260px; height: 370px; } h1 { color: #343f2f; font-family: Ringbearer, serif; font-position: center; font-size: 300%; margin: 0rem 0rem 0rem .5rem; text-shadow: 1px 1px 1px white; } h2 { font-family: Aniron, serif; text-align: center; text-shadow: .4px .4px .4px white; color: #7C0F01; margin: 0; padding: 0; } .row1 { display: inline-block; margin-top: 10px; margin-left: 15px; width: 630px; } .row2 { display: inline-block; margin-top: 10px; margin-left: 15px; width: 630px; } ul { list-style-type: none; color: white; margin: 0 0 0 0; border: 0 0 0 0; padding: 0 0 0 0; } li { background-color: #4f4722; font-family: MorrisRoman-Black, serif; color: white; opacity: .80; display: inline-block; text-align: center; min-width: 6rem; max-width: 6rem; padding: 0rem 0rem 0rem 0rem; border: 1px solid; border-radius: 10px; box-shadow: 2px 2px 2px black; border-color: white; margin: 0 5px 0 0; } } li:hover { background-color: #F4F4E9; } li:active { background-color: white; box-shadow: .80px .80px .80px #782320; transform: translateY(4px); } /* To convert cursor to pointer */ #aragorn, #faramir, #gimli, #frodo, #boromir, #pippin, #theoden, #samwise, #gandalf, #legolas, #merry, #treebeard { cursor: pointer; padding: 1rem; } /* HELP container */ .popup { font-family: Aniron, serif; font-size: 70%; box-shadow: 2px 2px 2px black; display: inline-block; float: right; cursor: pointer; background-color: #c3b291; margin-top: 30px; margin-right: 15px; border: 1px; border-radius: 10px; padding: 5px; } /* The actual popup (appears on top) */ .popup .popuptext { font-size: 70%; visibility: hidden; width: 579px; background-color: #243022; color: #fff; text-align: left; border-radius: 6px; padding: 15px 15px 15px 15px; position: absolute; z-index: 1; top: 6.5%; left: 38.5%; } /* Popup arrow */ .popup .popuptext: after { content: ""; margin-left: 5px; border-width: 5px; border-style: solid; border-color: #555 transparent transparent transparent; } /* Toggle this class when clicking on the popup container (hide and show the popup) */ .popup .show { visibility: visible; -webkit-animation: fadeIn 1s; animation: fadeIn 1s } .popup:hover { background-color: #F4F4E9; } /* Add animation (fade in the popup) */ @-webkit-keyframes fadeIn { from { opacity: 0; } to { opacity: 1; } } @keyframes fadeIn { from { opacity: 0; } to { opacity: 1; } }
Javascript Code Sample
The most important piece of the zodiac generator is JS code; without it, the page doesn’t function. It was here, over the course of 10 weeks, that I slowly created pieces of the code, building a foundation of functionality. I started first with the creation of three basic variables that would store values for the user’s first and last name, and the year they were born: var firstName, var lastName and var birthDayObj. I also created var weekDays, in which I immediately specified the days of the week.
Next, would come basic functionality, which would allow these variables to execute some form of action. In this case I needed to display a message once the user inputed the required data, i.e., their name and birthdate. So I created a function that would essentially convert these variables into values that could be processed, Within this function I created more variables that would request the current year, month and date from the computer. This would be eventually broken down into two separate functions: one would calculate the users age when they inputed their birthdate, while the other, using if statements, would find and display a particular zodiac based off a set perimeter of date ranges within the year. You can see a full breakdown of the code below.
// Declare global variables outside of functions here var firstName; var birthDayObj; // var WeekDays = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']; // var lastName; function fade2Content() { console.log('fade2Content called'); document.body.classList.remove("whiteScreen"); } function calZodiac() { // // Name and date error checking not needed when using Chrome browser firstName = document.getElementById('fname').value; birthDayObj = new Date(document.getElementById('bday').value); // // DATE CHECKER FOR SAFARI! This check will NOT catch Feb 30 console.log('birthDayObj parsed is ' + Date.parse(birthDayObj)); if (isNaN(Date.parse(birthDayObj))) { alert('Invalid date. Please retry'); // Exit/Jump out of this function return false; } var whichDayNdx = birthDayObj.getUTCDay(); console.log('whichDayNdx is ' + whichDayNdx); // // Calculate zodic sign var whichMonth = birthDayObj.getUTCMonth(); console.log('whichMonth is ' + whichMonth); var whichDayOfMonth = birthDayObj.getUTCDate(); console.log('whichDayOfMonth is ' + whichDayOfMonth); // // Add 1 for readabilty whichMonth++; whichDayOfMonth++; var AstroSign; if ((whichMonth == 12 && whichDayOfMonth >= 22) || (whichMonth == 1 && whichDayOfMonth <= 19)) { AstroSign = "treebeard"; displayZodSign('click', AstroSign); } else if ((whichMonth == 11 && whichDayOfMonth >= 22) || (whichMonth == 12 && whichDayOfMonth <= 21)) { AstroSign = "merry"; displayZodSign('click', AstroSign); } else if ((whichMonth == 10 && whichDayOfMonth >= 24) || (whichMonth == 11 && whichDayOfMonth <= 21)) { AstroSign = "legolas"; displayZodSign('click', AstroSign); } else if ((whichMonth == 9 && whichDayOfMonth >= 23) || (whichMonth == 10 && whichDayOfMonth <= 23)) { AstroSign = "gandalf"; displayZodSign('click', AstroSign); } else if ((whichMonth == 8 && whichDayOfMonth >= 23) || (whichMonth == 9 && whichDayOfMonth <= 22)) { AstroSign = "Samwise"; displayZodSign('click', AstroSign); } else if ((whichMonth == 7 && whichDayOfMonth >= 23) || (whichMonth == 8 && whichDayOfMonth <= 22)) { AstroSign = "theoden"; displayZodSign('click', AstroSign); } else if ((whichMonth == 6 && whichDayOfMonth >= 22) || (whichMonth == 7 && whichDayOfMonth <= 22)) { AstroSign = "pippin"; displayZodSign('click', AstroSign); } else if ((whichMonth == 5 && whichDayOfMonth >= 21) || (whichMonth == 6 && whichDayOfMonth <= 21)) { AstroSign = "borormir"; displayZodSign('click', AstroSign); } else if ((whichMonth == 4 && whichDayOfMonth >= 20) || (whichMonth == 5 && whichDayOfMonth <= 20)) { AstroSign = "frodo"; displayZodSign('click', AstroSign); } else if ((whichMonth == 3 && whichDayOfMonth >= 21) || (whichMonth == 4 && whichDayOfMonth <= 19)) { AstroSign = "gimli"; displayZodSign('click', AstroSign); } else if ((whichMonth == 2 && whichDayOfMonth >= 19) || (whichMonth == 3 && whichDayOfMonth <= 20)) { AstroSign = "faramir"; displayZodSign('click', AstroSign); } else if ((whichMonth == 1 && whichDayOfMonth >= 20) || (whichMonth == 2 && whichDayOfMonth <= 18)) { AstroSign = "aragorn"; displayZodSign('click', AstroSign); } // // Figure out user age, call a function, pass parameters, return result //var currAge = calculate_age(birthDayObj.getUTCMonth(), birthDayObj.getUTCDate(), birthDayObj.getUTCFullYear()); var outputArea = document.getElementById('displayMsg'); var msg = ''; msg += '<p>Hello, ' + firstName + '.</p>'; msg += '<p>In Middle Earth you would be known as ' + AstroSign; '.</p>'; msg += '</p>'; //outputArea.innerHTML = msg; // // return false to prevent form from resetting return false; } function calculate_age(bMonth, bDay, bYear) { var today_date = new Date(); var today_year = today_date.getUTCFullYear(); var today_month = today_date.getUTCMonth(); var today_day = today_date.getUTCDate(); console.log('bMonth is ' + bMonth); console.log('today_month is ' + today_month); console.log('bDay is ' + bDay); console.log('today_day is ' + today_day); console.log('bYear is ' + bYear); console.log('today_year is ' + today_year); var age = today_year - bYear; console.log('age is ' + age); if (today_month < bMonth) { age--; } if ((today_month == bMonth) && (today_day < bDay)) { age--; } console.log('adjusted age is ' + age); return age; } // Close calculate_age // Middle Earth Character Variables var treebeardObj = document.getElementById('treebeard'); var merryObj = document.getElementById('merry'); var legolasObj = document.getElementById('legolas'); var gandalfObj = document.getElementById('gandalf'); var samwiseObj = document.getElementById('samwise'); var theodenObj = document.getElementById('theoden'); var pippinObj = document.getElementById('pippin'); var boromirObj = document.getElementById('boromir'); var frodoObj = document.getElementById('frodo'); var gimliObj = document.getElementById('gimli'); var faramirObj = document.getElementById('faramir'); var aragornObj = document.getElementById('aragorn'); // alltxtObj / alltxtObj for getting Element by ID name (CSS) var alltxtObj = document.getElementById('alltxt'); var allPicsObj = document.getElementById('allpics'); // Charcter Var Event listeners to display on click treebeardObj.addEventListener('click', function (evt) { displayZodSign(evt, 'treebeard'); }, false); merryObj.addEventListener('click', function (evt) { displayZodSign(evt, 'merry'); }, false); legolasObj.addEventListener('click', function (evt) { displayZodSign(evt, 'legolas'); }, false); gandalfObj.addEventListener('click', function (evt) { displayZodSign(evt, 'gandalf'); }, false); samwiseObj.addEventListener('click', function (evt) { displayZodSign(evt, 'samwise'); }, false); theodenObj.addEventListener('click', function (evt) { displayZodSign(evt, 'theoden'); }, false); pippinObj.addEventListener('click', function (evt) { displayZodSign(evt, 'pippin'); }, false); boromirObj.addEventListener('click', function (evt) { displayZodSign(evt, 'boromir'); }, false); frodoObj.addEventListener('click', function (evt) { displayZodSign(evt, 'frodo'); }, false); gimliObj.addEventListener('click', function (evt) { displayZodSign(evt, 'gimli'); }, false); faramirObj.addEventListener('click', function (evt) { displayZodSign(evt, 'faramir'); }, false); aragornObj.addEventListener('click', function (evt) { displayZodSign(evt, 'aragorn'); }, false); // Function for displaying Variables when called function displayZodSign(evt, whichOne) { console.log('displayZodSign called ' + whichOne); var imgArray = allPicsObj.children; console.log('imgArray is ' + imgArray); console.log('imgArray length is ' + imgArray.length); console.log('imgArray 2 is ' + imgArray[2].tagName); // Loop through array and remove display class from everything for (lp = 0; lp < imgArray.length; lp++) { imgArray[lp].classList.remove('display'); } // For Loop Closed msg = 'Hello World'; switch (whichOne) { case 'treebeard': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Treebeard (Ent)<p>Dec 22 - Jan 19<br>Also known as Fangorn, the oldest of the ents, shepards of the trees. An ancient tree-like being who resided in Fangorn Forest, and taking no part in the affairs of Men, Elves and Wizards. However, Treebeard was brought into the War of the Ring once Saruman the White began cutting down the forest to power his war effort. An angry Treebeard called all the ents together to attack Isengard, in an event known as the Last March of the Ents."; imgArray[12].classList.add('display'); playAudio('treebeardAudio'); break; case 'merry': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Meriadoc Brandybuck (Hobbit)<p>Nov 22 - Dec 21<br>Lived in: Brandy Hall, Buckland<br>Meriadoc, or Merry, Brandybuck, son of Saradoc Brandybuck and Esmeralda Took, was a Hobbit of the Shire. He rose to fame as one of the members of the Fellowship of the Ring. After the War of the Ring ha was called The Magnificent and became the Master of Buckland after his father."; imgArray[11].classList.add('display'); playAudio('merryAudio'); break; case 'legolas': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Legolas (Elf, Sindar)<p>Oct 24 - Nov 21<br>Legolas was a member of the Fellowship of the Ring. His father was Thranduil, King of the Elves of Northern Mirkwood. He represented his father at the Council of Elrond and became one of the Nine Walkers. He departed from the Ring-bearer at Amon Hen, and was later involved in the Battle at Helms Deep, the Battle of the Pelennor Fields, and the Battle of the Morannon."; imgArray[10].classList.add('display'); playAudio('legolasAudio'); break; case 'gandalf': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Gandalf the Grey (Istar, wizard)<p>Sep 23 - Oct 23<br>Later known as Gandalf the White, also named originally Olórin, was sent from the West in the Third Age to combat the threat of Sauron. He joined Thorin and his company to reclaim the Lonely Mountain from Smaug, the Dragon; convoked the Fellowship of the Ring to destroy the One Ring, and led the Free Peoples in the final campaign of the War of the Ring."; imgArray[9].classList.add('display'); playAudio('gandalfAudio'); break; case 'samwise': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Samwise Gamgee (Hobbit)<p>Aug 23 - Sep 22<br>Also known as Sam, of the Shire. He was Frodo Baggins, The Ring-Bearer's, gardener and best friend. Sam proved himself to be Frodo's closest and most dependable companion during the War of the Ring. The most loyal member of the Fellowship of the Ring, and only one to remain at Frodo's side to the end of the journey to destroy the One Ring of Power in fires of Mount Doom."; imgArray[8].classList.add('display'); playAudio('samwiseAudio'); break; case 'theoden': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Theoden, King of Rohan (Human)<p>Jul 23 - Aug 22<br>Theoden, son of Thengel and Morwen, and the seventeenth King of Rohan. Theoden defended Rohan from the forces of Saruman in the Battle of Helm's Deep and subsequently led a host to the aid of Gondor in the Battle of Pelennor Fields. During the battle he was mortally wounded by the Witch-king of Angmar."; imgArray[7].classList.add('display'); playAudio('theodenAudio'); break; case 'pippin': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Peregrin Took (Hobbit)<p>Jun 22 - Jul 22<br>More commonly known as Pippin, a Hobbit of the Shire, and one of Frodo Baggins, The Ring-bearer's youngest, but closest friends. He was a member of the Fellowship of the Ring. After the Fellowship fractured at Amon Hen, Pippin, along with Merry, found themselves lost in Fangorn Forest where they met Treebeard, and were intergral in convinving the Ent to take up arms against Saruman and his evil mechinations."; imgArray[6].classList.add('display'); playAudio('pippinAudio'); break; case 'boromir': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Boromir, Steward Prince of Gondor (Human)<p>May 21.. Jun 21<br>Was a valiant warrior known in Gondor for his greatness, having already achieved great merit in Gondor prior to the Council of Elrond, where he joined the Fellowship of the Ring. He was the eldest son of Denethor II, Steward of Gondor during the War of the Ring, and brother to Faramir. Boromir gave is life defending Hobbits, Merry and Pippin during the Battle o Amon Hen."; imgArray[5].classList.add('display'); playAudio('boromirAudio'); break; case 'frodo': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Frodo Baggins, The Ring-bearer, (Hobbit)<p>Apr 20 - May 20<br>Arguably the most important and most famous Hobbit in the history of Middle-earth for his involvement in the War of the Ring. He bore the One Ring to Mount Doom where it was destroyed and thus causing the final defeat of Sauron. He was also notable for being one of the three Hobbits, along with Samwise Gamgee and Bilbo Baggins, who sailed west to Aman, in the Undying Lands."; imgArray[4].classList.add('display'); playAudio('frodoAudio'); break; case 'gimli': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Gimli, Lord of the Glittering Caves (Dwarf)<p>Mar 21 - Apr 19<br>A well-respected dwarf warrior in Middle-earth during the Great Years. He was a member of the Fellowship of the Ring, and played a critical role in the defeat of the forces of Isengard at Helms Deep. Gimli was the only one of the dwarves to readily fight alongside elves in the war against Sauron at the end of the Third Age. After the defeat of Sauron, he was given lordship of the Glittering Caves at Helm's Deep."; imgArray[3].classList.add('display'); playAudio('gimliAudio'); break; case 'faramir': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Faramir, Prince of Ithilien (Human)<p>Feb 19 - Mar 20<br>The Captain of the Rangers of Ithilien and Captain of the White Tower of Gondor after his brother's death. Faramir was also the last Ruling Steward of Gondor. Upon the arrival of the true king, King Aragorn Elessar, he laid down his office, but Elessar renewed the hereditary appointment of Steward as the advisor to the King. After the War of the Ring, Faramir was also appointed the Prince of Ithilien."; imgArray[2].classList.add('display'); playAudio('faramirAudio'); break; case 'aragorn': // Display Big Image for Pis // displayObj.src = 'img/ful_pisces.png'; msg = "Aragorn Elessar, King of Gondor<p>Jan 20 - Feb 18<br>Also known as Strider; the true heir to the throne of Gondor; and a Chieftain of the Dunedain. At the end of the Third Age, Aragorn helped destroy the One Ring and defeat Sauron and subsequently reunited the Kingdoms of Arnor and Gondor. He was part of the Fellowship of the Ring, and along with Gimli, Legolas and Gandolf helped defend Helms Deep, and came to the aid of Gondor and Rohan forces during the battle of Pelennor Fields."; imgArray[1].classList.add('display'); playAudio('aragornAudio'); break; default: // displayObj.src = 'img/ful_blank.png'; imgArray[0].classList.add('display'); } // Switch End alltxtObj.innerHTML = msg; } // Function Closed // When the user clicks on <div>, open the Help Menu function myFunction() { var popup = document.getElementById("myPopup"); popup.classList.toggle("show"); } // Function Closed function playAudio(whichSound) { // play sound based on whichSound par document.getElementById('aragornAudio').pause(); document.getElementById('aragornAudio').currentTime = 0.0; document.getElementById('faramirAudio').currentTime = 0; document.getElementById('faramirAudio').pause(); document.getElementById('gimliAudio').currentTime = 0; document.getElementById('gimliAudio').pause(); document.getElementById('frodoAudio').currentTime = 0; document.getElementById('frodoAudio').pause(); document.getElementById('boromirAudio').currentTime = 0; document.getElementById('boromirAudio').pause(); document.getElementById('pippinAudio').currentTime = 0; document.getElementById('pippinAudio').pause(); document.getElementById('theodenAudio').currentTime = 0; document.getElementById('theodenAudio').pause(); document.getElementById('samwiseAudio').currentTime = 0; document.getElementById('samwiseAudio').pause(); document.getElementById('gandalfAudio').currentTime = 0; document.getElementById('gandalfAudio').pause(); document.getElementById('legolasAudio').currentTime = 0; document.getElementById('legolasAudio').pause(); document.getElementById('merryAudio').currentTime = 0; document.getElementById('merryAudio').pause(); document.getElementById('treebeardAudio').currentTime = 0; document.getElementById('treebeardAudio').pause(); document.getElementById(whichSound).play(); }
Conclusion
By finishing this project, I was able to gain a fundamental understanding of how JS works. I worked through the process, step-by-step and line-by-line of code. Initially, I was unsure if I could do this project correctly, as there is so much much more involved with JS than with HTML and CSS. What helped me along the way was keeping in mind three things: one, with JS, it is even more important where your code is placed, because if not in it’s correct spot, it will break the webpage; this ties into the second thing, keep your code clear, clean and concise; finally, go slow. I learned to be a lot more methodical in my coding practices because of this project. I am quite proud of it. Go ahead and check it out for yourself below.